Django Template

A Django template is a text document or a Python string marked-up using the Django template language. Some constructs are recognized and interpreted by the template engine. The main ones are variables and tags. A template is rendered with a context.
Click here Django template documentation
Django templates: Create your First Template in easy steps
The third and most significant aspect of Django’s MVT Structure is templates. In Django, a template is a .html file that is prepared in HTML, CSS, and Javascript. The Django framework efficiently manages and generates dynamically generated HTML web pages for end-user viewing. Django is mostly a backend framework, thus we use templates to offer a frontend and a layout for our website.
All static objects in a web page developed with Django hold the templates for generating the static entities, which are important to understand and run the process of Django applications. In other words, the templates are in charge of constructing the skeleton of a webpage. These templates allow you to set up an MVT-oriented architecture in Django. A Django view is used to render the templates used in a Python setup.
Need of Templates in Django
We can’t put python code in an HTML file since the code is only interpreted by the Python interpreter, not the browser. HTML is a static markup language, but Python is a dynamic programming language.
The Django template engine separates the design from the python code, allowing us to create dynamic web pages.
Rendering Django Views using Templates.
Given below are the templates to render Django views
1. Create a template folder
All template related HTML code could be placed into this folder. The template folder has to be created under the primary project folder.
2. Tag the template folder in settings.py file
The settings.py file is used for tagging these templates to their associated views. When the tagging process is accomplished it allows all the HTML contents placed inside the folder to be falling under this template section.
Code:
import os
# Build paths inside the project like this: os.path.join(BASE_DIR,...)
BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
Template_DIR = os.path.join(BASE_DIR,'Templates')
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [Template_DIR,],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
,
},
},
]Template_DIR = os.path.join(BASE_DIR,'Templates')
For implementing the Django template’s API backend the python path in the BACKEND key is used. Some among the backends which are built in Django are django.template.backends.django.DjangoTemplates and django.template.backends.jinja2.Jinja2.
- The directory at which the template engine needs to locate the template related search files is placed in the DIRS directory.
- Whereas the APP_DIRS is helpful in mentioning the location at which the engine has to verify the templates within the installed applications. For every subdirectory inside the applications a conventional name is provided by each defined backend.
3. Place the HTML file inside the templates folder
Code:
<!DOCTYPE html>
<html lang=”en” dir=”ltr”>
<head>
<meta charset=”utf-8″>
<title>Django App1</title>
</head>
<body>
<h1> Hello world from HTML page <h1>
</body>
</html>
Syntax:
render(request,template_name,context=None,content_type=None,status=None,using=None)
.arguments | Description |
request | This is used to generate a response. This is a mandatory argument. |
template name | Name of the template used for this view. This is a mandatory argument. |
context | A context is a variable name and variable value mapping maintained as a dictionary. In default,this is an empty dictionary. So if the key is passed the corresponding value from the dictionary can be retrieved and rendered. This is an optional argument. If nothing is provided for the context then render a empty context. |
content_type | MIME(Multipurpose Internet Mail Extensions) to be used. The default value is ‘text/html’. This is a optional argument. |
status | The response code to be used. The default response code is 200. |
using | This argument is used to represent the name of the template engine used for loading the template. This is a optional argument. |
Example:
Code:
from django.shortcuts import render
from django.http import HttpResponse
def index(request_iter):
return render(request_iter,’design.html’)
4. Tag the view in urls.py file
This is the process of creating a url for the view. The url mentioned here will be used for reaching the web page which is been mentioned.
- Import the library from django.conf.urls import url.
- declare a url entry in the urlpatterns list.
url(url_path,view_to_be_tagged,name_for_this_view)
from django.contrib import admin
from django.conf.urls import url
from Django_app1 import views
urlpatterns = [
url(r’^$’,views.index,name=’index’),
url(r’admin/’,admin.site.urls),]
5. Reload the server using python manage.py runserver command and verify webpage
The system message which is been placed below will be printed in the project console on loading the runserver. This message holds detailed information of when the server was started, the Django version which is been used and the http link at which the server is kickstarted.
Code:
Watching for file changes with StatReloader
Performing system checks…
System check identified no issues (0 silenced).
June 10, 2020 – 11:23:00
Django version 3.0.7, using settings ‘filename.settings’
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
Output:
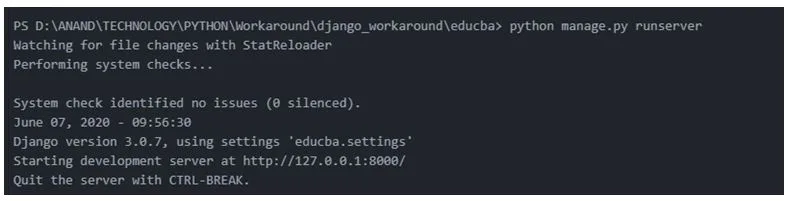
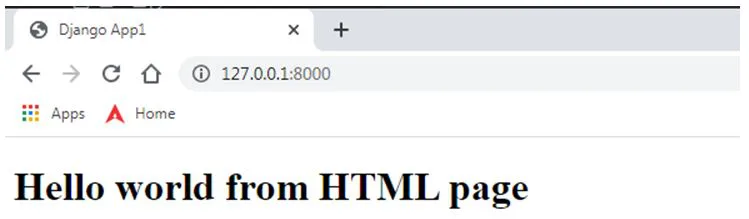
Django View Rendered from Template Tag
Given below are the Django view rendered from template tag:
1. The template tags are used for injecting dynamically generated content to the Django views. This is among the key functionalities of template tag. They could flexibly inject dynamic contents into the file.
The template tag filters could use the below listed options:
All Options in Template Filters
add | addslashes | capfirst |
center | cut | date |
default | dictsort | divisibleby |
escape | filesizeformat | first |
join | last | length |
linenumbers | lower | make_list |
random | slice | slugify |
time | timesince | title |
unordered_list | upper | wordcount |
2. In this example we have added the if tags and the filter tags to the template.
- Add a template tag in the HTML file.
Code:
<!DOCTYPE html>
<html lang=”en” dir=”ltr”>
<head>
<meta charset=”utf-8″>
<title>Django App1</title>
</head>
<body>
<h1> <u> All valid Technical tutorials </u> </h1>
{% if Entity_type == ‘tutorial’ %}
{{ Entity_name }}
{% else %}
{{ Error_Message }}
{% endif %}
<h2> <u> Django filters Explained <u> </h2>
<p> Student Count: {{ Entity_students_count | add:”230″}} <p>
<p> Entity Type: {{ Entity_type | capfirst}} <p>
</body>
</html></html></html>
Example:
from django.shortcuts import render
from django.http import HttpResponse
def index(request_iter):
dict_Var = {
“Entity_name”: “Educba”,
“Entity_type”: “tutorial”,
“Entity_students_count”: 345,
“Error_Message”: “No Valid Entity found”
}
return render(request_iter,’design.html’,context=dict_Var)>
3. Reload the server using python manage.py runserver command and verify the webpage.
Output:
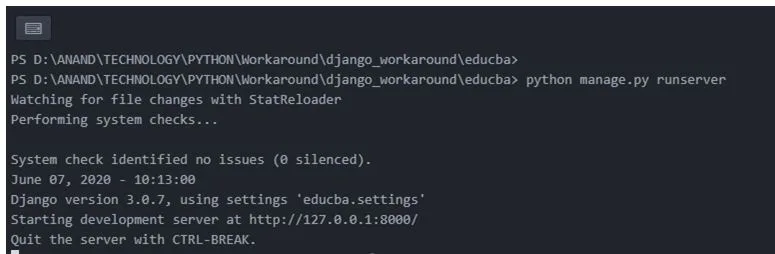
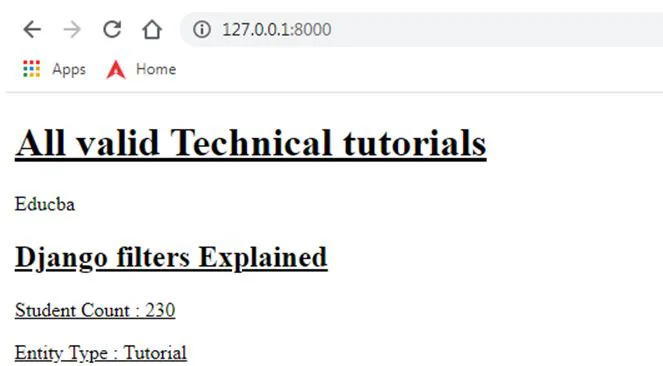
Conclusion
The use of templates is on among the biggest capabilities of Django in web development framework. These templates allow the Django setup to flexibility transfer dynamic content between the front end and the middle stream.