Introduction
In today’s interconnected world, catering to a diverse user base is crucial for the success of any web application. Django, the popular Python web framework, offers robust internationalization (i18n) and localization (l10n) features to help developers create multilingual and culturally adapted websites. In this comprehensive guide, we will explore the concepts of internationalization and localization in Django, understand their importance, and delve into practical examples to showcase their implementation.
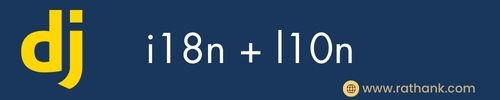
1. Understanding Internationalization and Localization
Internationalization involves designing a website to support multiple languages, while localization focuses on adapting the content to specific regions or cultures. Django provides powerful tools for achieving these goals.
2. Preparing Your Django Project for Internationalization
To enable internationalization, configure your Django project by updating the settings.py
file with the necessary language-related settings, middleware, and context processors.
# settings.py LANGUAGE_CODE = 'en-us' TIME_ZONE = 'UTC' USE_I18N = True USE_L10N = True
3. Translating Text Strings
Django uses translation catalogs and template tags to handle translations. Begin by marking translatable strings in templates and Python code.
# views.py from django.utils.translation import gettext as _ def home(request): greeting = _("Welcome to our website!") return render(request, 'home.html', {'greeting': greeting})
Extract the translation strings using Django’s management commands:
$ python manage.py makemessages -l es
Translate the extracted strings in the generated .po
file:
# es.po msgid "Welcome to our website!" msgstr "¡Bienvenido a nuestro sitio web!"
4. Handling Date, Time, and Number Formats
Django provides localization settings to handle date, time, and number formats according to each locale.
# settings.py DATE_FORMAT = 'd/m/Y' TIME_FORMAT = 'H:i' DECIMAL_SEPARATOR = ','
Use Django’s template filters and formatting functions for localized output:
<!-- home.html --> {{ some_date|date }} {{ some_number|floatformat }}
5. Implementing Language Switching
Allow users to switch between languages by configuring URL patterns or session-based language preferences.
# urls.py from django.conf.urls import i18n urlpatterns = [ path('i18n/', include('django.conf.urls.i18n')), # Other URL patterns ]
Implement language dropdowns or flags for user selection:
<!-- language_dropdown.html --> <form action="{% url 'set_language' %}" method="post"> {% csrf_token %} <input name="next" type="hidden" value="{{ request.path }}">; <select name="language"> {% get_language_info_list for LANGUAGES as languages %} {% for language in languages %} <option value="{{ language.code }}" {% if language.code == LANGUAGE_CODE %}selected{% endif %}> {{ language.name_local }} </option> {% endfor %} </select> <button type="submit">Go</button> </form>
6. Customizing Translations
Override translations for specific phrases or sections, handle plurals, and language-specific grammatical rules.
# es.po msgid "Welcome to our website!" msgid_plural "Welcome to our websites!" msgstr[0] "¡Bienvenido a nuestro sitio web!" msgstr[1] "¡Bienvenido a nuestros sitios web!"
Use translation placeholders for dynamic content:
# views.py from django.utils.translation import gettext as _ def profile(request): username = request.user.username message = _("Hello, %(username)s!") % {'username': username} return render(request, 'profile.html', {'message': message})
7. Managing Translations with Django’s Admin Interface
Leverage Django’s admin interface for translation management, allowing translators to update translations easily.
# admin.py from django.contrib import admin from django.utils.translation import gettext_lazy as _ @admin.register(YourModel) class YourModelAdmin(admin.ModelAdmin): list_display = ('name', 'translated_field') def translated_field(self, obj): return _(obj.field_name) translated_field.short_description = _('Translated Field')
8. Testing and Debugging Internationalized Applications
Write tests for internationalized features and use Django’s language tools for debugging and testing.
# tests.py from django.test import TestCase from django.utils.translation import activate class MyTest(TestCase): def test_home_view(self): activate('es') response = self.client.get('/') self.assertContains(response, '¡Bienvenido a nuestro sitio web!')
9. Dealing with Right-to-Left Languages
Support languages with right-to-left (RTL) writing systems by ensuring proper bidirectional text and layout adjustments.
10. SEO Considerations for Multilingual Websites
Optimize multilingual websites by setting language-specific URLs, hreflang tags, handling duplicate content, and implementing language-specific metadata.
Conclusion
By harnessing Django’s internationalization and localization capabilities, developers can create powerful multilingual websites that cater to a global audience. From translating text strings to handling date formats and implementing language switching, Django empowers you to unlock new horizons and provide exceptional user experiences worldwide.
By following this ultimate guide, you’ll be well-equipped to create internationalized and localized Django applications that open doors to global opportunities.
Happy coding and expanding your user base!