from django.shortcuts import render from .forms import RegistrationForm from .models import Account # Create your views here. def register(request): # Checking if POST request comes in if request.method == 'POST': # getting all the data from the form using POST request form = RegistrationForm(request.POST) # checking if the data are valid or not if form.is_valid(): # taking out all data from each inputs. as we are using forms, we need to use .cleaned_data first_name = form.cleaned_data['first_name'] last_name = form.cleaned_data['last_name'] email = form.cleaned_data['email'] password = form.cleaned_data['password'] phone_number = form.cleaned_data['phone_number'] # creating username by spliting the email and taking part before the @ username = email.split('@')[0] # Creating the user object with the data we got. # Here, we are using the create_user() method of MyAccountManager class using the Account class, # As MyAccountManager class is used as object in Account class user = Account.objects.create_user(first_name=first_name, last_name=last_name, username=username, email=email, password=password) # assigning the phone_number to the user as phone_number is not a field in create_user() method user.phone_number = phone_number # saving the user user.save() form = RegistrationForm() context = { 'form' : form, } return render(request, 'accounts/register.html', context)
The error you're encountering is due to the phone
field in your Account
model being set to unique=True
. This requires each phone number to be unique, but you may be trying to use the same number twice, or possibly leaving it blank when creating a user.
To resolve this, you should remove unique=True
from the phone
field in your Account
model. Instead, handle the uniqueness check in your forms.py
. This way, you can verify if the phone number is already taken and display an appropriate message to the user.
If you still experience issues after making these changes, please share your updated GitHub repository, so we can provide more accurate assistance.
Let me know if there's anything else I can help with!
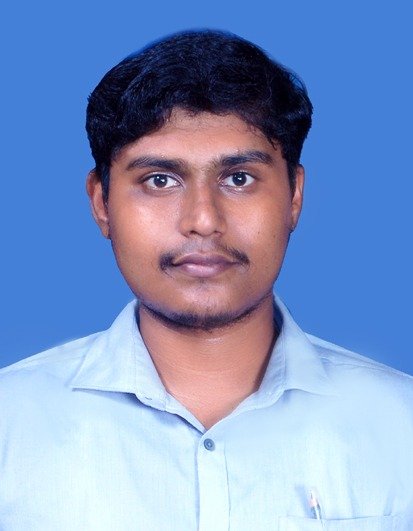
@mohaiminul-islam While creating the super user I have not provided the phone number. Hence while creating a normal user, I was getting the error. Thank you for your help. I removed unique=True and made blank=True. Thank you for your help.